Reading a folder with unknown number of files in Java
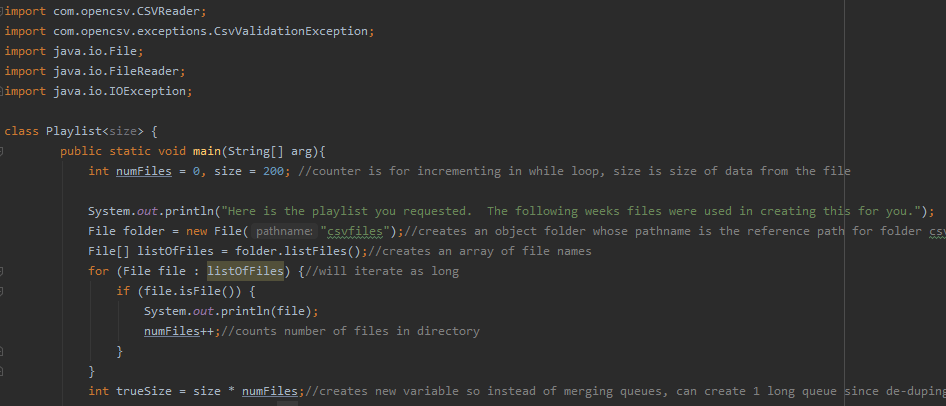
One step of my second assignment in constructing a playlist involved reading in a list of csv files to construct the playlist from. I wanted to make a pretty comprehensive way to do it without listing all the files in the program incase we had to revisit this for the final assignment, or if I just wanted to reuse it for something else later on. So I went to look at the java docs for the File class we usually work with to see all the options, which you can see here.
Looking at all the methods the most useful one is the listOfFiles method, which returns an array of all the file names with their relative paths. Assuming all files we want read are being placed in a folder in the project directory called 'csvfiles', an example of this in use can be seen below.
File folder = new File("csvfiles");
File[] listOfFiles = folder.listFiles();
First line creates an object called folder of type File, and it's referencing the relative path of the csvfiles folder. Second line creates an array of objects called listofFiles of type File, and the listFiles method will return all the files in the folder and save it to the array. But let's say you want to either output all the files that are being read, or want to verify that the files are being read correctly.
int numFiles = 0;
for (File file : listOfFiles) {
if (file.isFile()) {
System.out.println(file);
numFiles++;//counts number of files in directory
}
}
We create an object file of type File, that will iterate as long as the array listOfFiles has values in it(in my case it will go 5 times). The isFile() method is essentially a boolean check, if it reads a proper file path it will execute, if not then it skips the if loop. We print out the file path and then with a counter variable created before the for loop we count the number of files total that are being read. Sample output for just this step should look something like this
csvfiles\regional-global-weekly-2020-01-17--2020-01-24.csv
csvfiles\regional-global-weekly-2020-01-24--2020-01-31.csv
csvfiles\regional-global-weekly-2020-01-31--2020-02-07.csv
csvfiles\regional-global-weekly-2020-02-07--2020-02-14.csv
csvfiles\regional-global-weekly-2020-02-14--2020-02-21.csv
I'm using the same sets of data that I talked about in my last post but including more than just the 1-17 to 1-24 sets of streaming data from Spotify. From here I've validated the listofFiles array is valid and I can either use that to create a Queue for each one to later merge , or I can create a large Queue whose total size is that of all the files times the number of datasets per file which in the case of spotify csv's are 200.
int size = 200;//Total number of songs in a spotify csv file
int trueSize = size * numFiles;//equal to 1000
The counter numFiles is not necessary depending on how you are looking to execute the reading of all the files into Queues, but it's an option and one to keep in mind depending on exactly what type of data you are looking to read and parse.